Abstract: Extend the custom architecture dependencies graph by adding clusters.
In a previous article, we made an architecture dependency graph with custom edges. However, the graph doesn’t preserve parent-children relationships. For example, if you made this architecture in Fastgrep:
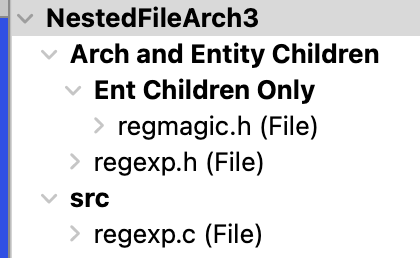
You’d get this graph:
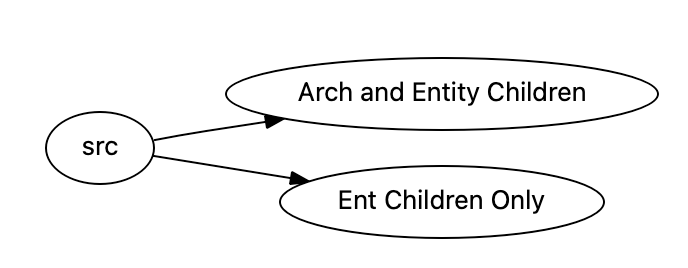
But, “Ent Children Only” is a child of “Arch and Entity Children.” A parent child relationship is usually shown by creating clusters. This article describes how to extend the previously described plugin to cluster architectures.
Python Code
First, we’ll update the name of the graph:

Next, we need to track the created clusters just like we track the created nodes. So, we’ll have a clusters dictionary in the draw function that gets passed to grabNode:
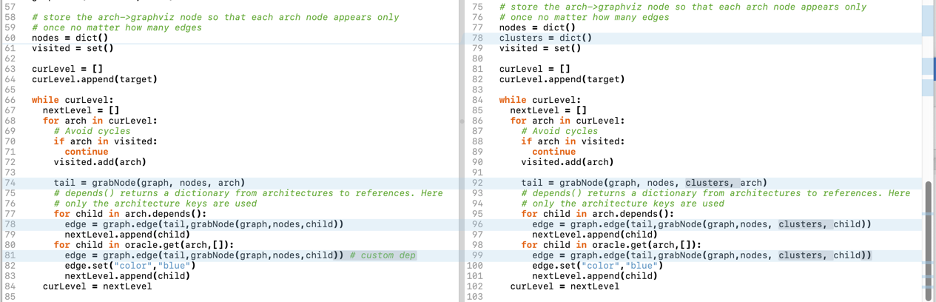
Then, we need a grabCluster function that will create new clusters as needed. The grabCluster function must be recursive to allow an arbitrary nesting level. The recursion ends when arch is “None”, meaning there was no parent. In that case, the graph object is returned.
def grabCluster(graph, clusters, arch):
if not arch:
return graph
if arch not in clusters:
parcluster = grabCluster(graph,clusters,arch.parent())
clusters[arch] = parcluster.cluster(arch.name(),arch)
return clusters[arch]
Finally, the grabNode function needs to be updated to use the correct graph parent for each node. There’s a little bit of trickiness here because, like the example at the beginning, an architecture might have both entity and architecture children. In that case, there might be an edge directly to the architecture and edges to its architecture children. We catch that case by checking if there are architecture children on the node we’re grabbing. If there are, we style this node as just text and clear the label on the cluster making it look like the edge goes to the cluster label.
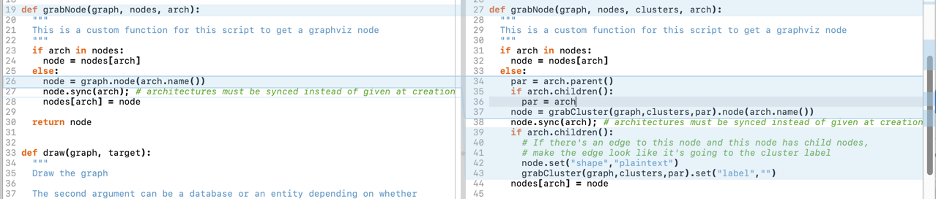
Now, with the initial architecture we get this graph:
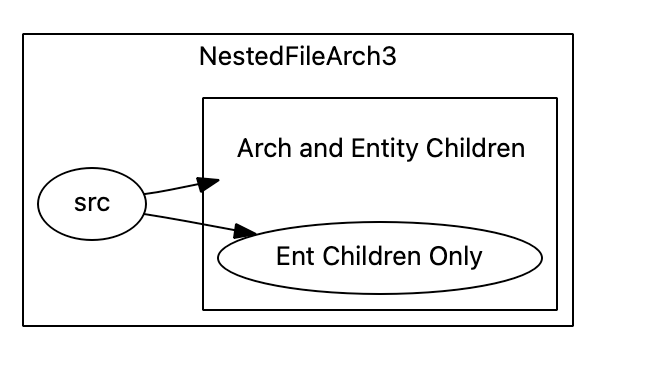